Introduction
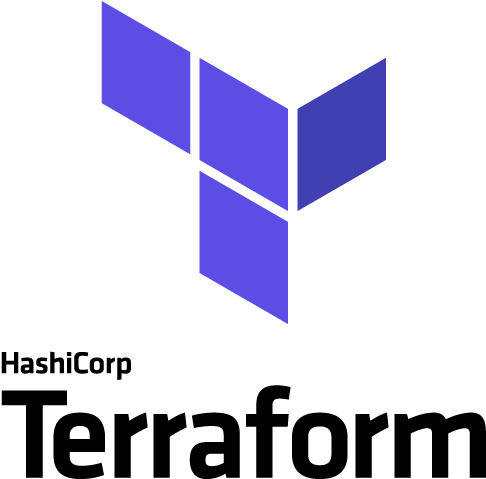
Terraform is a popular Infrastructure as Code (IaC) tool that helps you manage your infrastructure resources in a safe, predictable, and version-controlled manner. One of the key strengths of Terraform is its ability to interact with various cloud platforms and other infrastructure providers, such as Google Cloud, AWS, Azure, and others.
However, Terraform doesn’t have built-in support for every infrastructure provider out there, and sometimes you may need to interact with a custom API, an internal tool, or a legacy system that Terraform doesn’t support. This is where Terraform providers come in.
In this article, we’ll explore what Terraform providers are, why you might want to create one, and how to do it.
What is a Terraform Provider?
A Terraform provider is a piece of software that implements Terraform’s resource type definitions and implements the CRUD (Create, Read, Update, Delete) operations for those resource types. The provider acts as an intermediary between Terraform and the underlying infrastructure, allowing Terraform to interact with your resources.
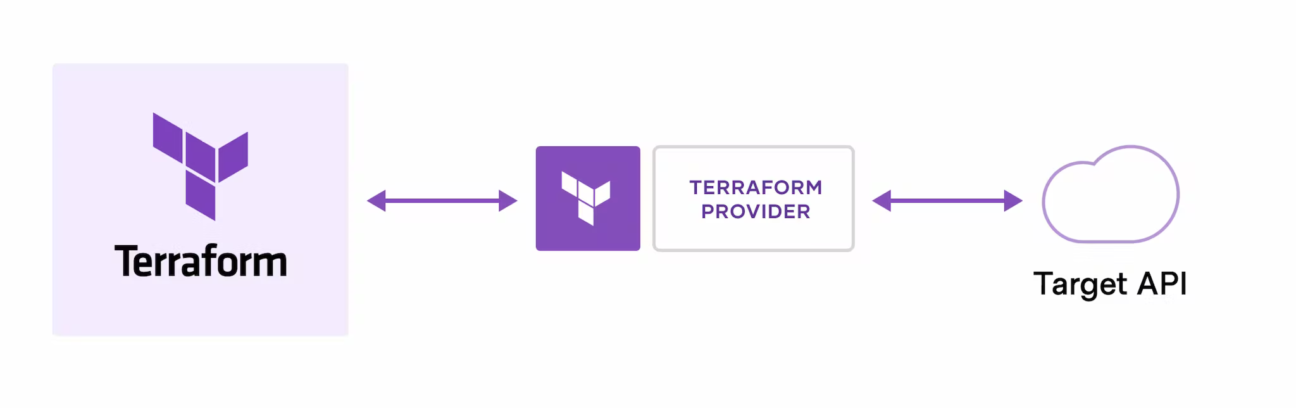
Why Create a Terraform Provider?
There are several reasons why you might want to create a Terraform provider:
- To support an infrastructure provider or service that Terraform doesn’t support natively.
- To manage custom APIs, internal tools, or legacy systems that Terraform doesn’t support.
- To simplify and standardize the management of infrastructure resources, reducing the risk of errors and making it easier to track changes.
- To streamline the automation of infrastructure management tasks, saving time and reducing manual effort.
How to Create a Terraform Provider
Creating a Terraform provider is a technical task that requires some programming knowledge. Here are the basic steps to create a Terraform provider:
- Choose a programming language: Terraform providers can be written in any programming language, but Go is the most common language used for creating Terraform providers.
- Decide on the resource types: Determine the types of resources you want to manage with your Terraform provider and the CRUD operations you want to implement for each resource type.
- Implement the provider: Write the code to implement the provider, including the resource types and the CRUD operations.
- Test the provider: Test the provider thoroughly to make sure it works as expected and doesn’t have any unintended consequences.
- Publish the provider: Publish the provider on a public or private registry, so that other users can install and use it.
Provider example
Looker is a business intelligence and data analytics platform that helps organizations effectively access, analyze, and visualize their data. With its web-based interface and customizable tools, Looker makes it easy for users to query, report, and explore data and create custom dashboards, charts, and reports.
However, for companies managing hundreds of projects on Looker, manually using the Looker user interface can be inefficient. This is where automation becomes crucial. Although Looker doesn’t offer an official provider, existing solutions online may not meet the specific needs of clients.
To address this challenge, we created our own Looker Terraform provider to better serve our clients. This provider is now open-source and available for free on both GitHub and the Terraform registry.
Technical tutorial
In this tutorial, we’ll create a simple example provider using Golang to help you understand the fundamental workings of a terraform provider.
Prerequisites
- Go programming language installed on your system.
- Terraform installed on your system.
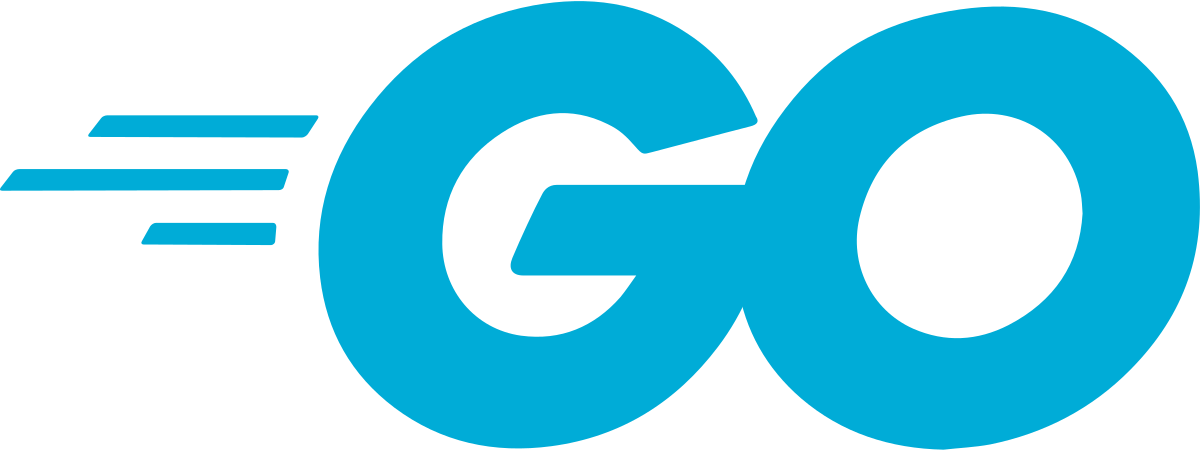
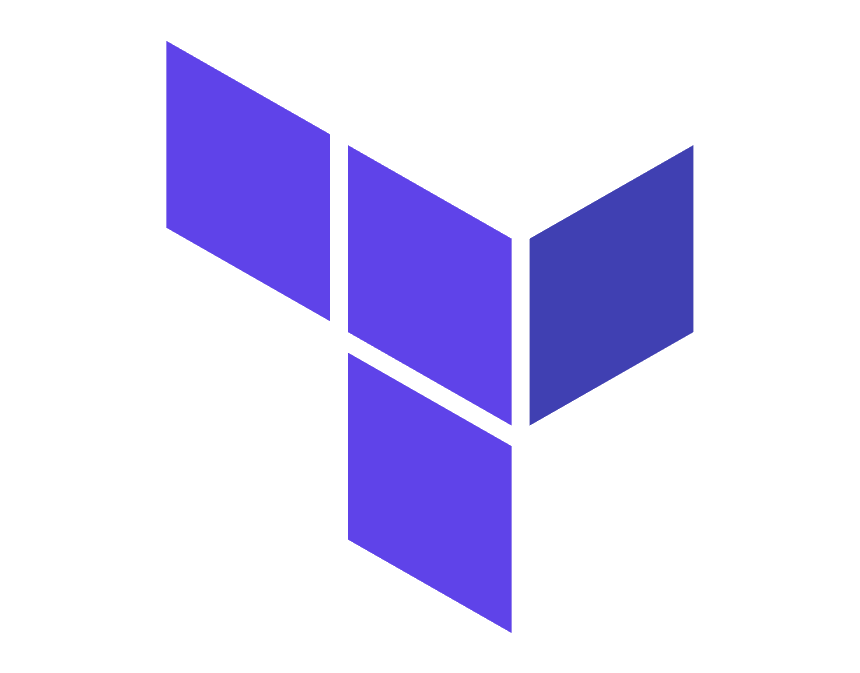
Create a new directory for your Terraform provider
Create a new directory for your Terraform provider project and navigate to it in your terminal.
Initialize a Go module
Run the following command in your terminal to initialize a Go module:
go mod init <module-name>
Define the provider schema
The provider schema defines the resources that the provider will manage and the input arguments for those resources. It is defined in a file named provider.go.
Create a new file named provider.go in your provider directory and add the following code:
package main
package main
import (
"github.com/hashicorp/terraform-plugin-sdk/helper/schema"
)
func Provider() *schema.Provider {
return &schema.Provider{
ResourcesMap: map[string]*schema.Resource{
"example_resource": resourceExample(),
},
}
}
In this example, the provider is defined to manage a single resource named example_resource.
Define the resource schema
The resource schema defines the input arguments and the actions that the Terraform provider will perform for the resource.
Add the following code to your provider.go file:
func resourceExample() *schema.Resource {
return &schema.Resource{
Schema: map[string]*schema.Schema{
"input_argument_1": &schema.Schema{
Type: schema.TypeString,
Required: true,
},
"input_argument_2": &schema.Schema{
Type: schema.TypeInt,
Optional: true,
},
},
Create: resourceExampleCreate,
Read: resourceExampleRead,
Update: resourceExampleUpdate,
Delete: resourceExampleDelete,
}
}
In this example, the resource schema is defined with two input arguments: input_argument_1 and input_argument_2. The four actions (Create, Read, Update, and Delete) that Terraform will perform for this resource are also defined.
Implement the resource actions
The resource actions are implemented as Go functions. Add the following code to your provider.go file:
func resourceExampleCreate(d *schema.ResourceData, meta interface{}) error {
// Create the resource
d.SetId("example_resource_id")
return nil
}
func resourceExampleRead(d *schema.ResourceData, meta interface{}) error {
// Read the resource
return nil
}
func resourceExampleUpdate(d *schema.ResourceData, meta interface{}) error {
// Update the resource
return nil
}
func resourceExampleDelete(d *schema.ResourceData, meta interface{}) error {
// Delete the resource
d.SetId("")
return nil
}
Finally, you can build the provider:
go build -o build/terraform-provider-<PROVIDER_NAME> .
Devoteam G Cloud expertise
Devoteam G Cloud is a highly skilled cloud engineering and consulting firm with a focus on cloud infrastructure and automation. We have a team of experts with extensive experience in creating Terraform providers and developing custom Cloud solutions. Our expertise in both Terraform and software development allows us to create high-quality, scalable, and flexible Terraform providers that meet the specific needs of our clients. Whether you need to manage a custom API, an internal tool, or a legacy system, Devoteam G Cloud has the skills and experience to deliver a Terraform provider that will help you manage your infrastructure resources more effectively. With a focus on delivering value to their clients and a commitment to quality, Devoteam G Cloud is a trusted partner for businesses seeking to optimize their infrastructure management with Terraform.
Creating a Terraform provider is a valuable way to extend Terraform’s capabilities and streamline the management of your infrastructure resources. By creating a Terraform provider, you can support custom APIs, internal tools, or legacy systems that Terraform doesn’t support natively, and standardize the management of your infrastructure resources, reducing the risk of errors and making it easier to track changes. With some programming knowledge and effort, you can create a Terraform provider that meets your specific needs and helps you manage your infrastructure resources more effectively.
Streamline your Infrastructure Management
Optimize your Cloud Infrastructure with our expertise in Terraform and Custom Cloud Solutions.